Web Cookies allow you to preserve the session information from one page load to another. So often web developers need to be able to set and unset cookies on their web pages. In this article, we will learn how to set/unset cookie in jQuery. Although it can be done using plain JavaScript also, it is much easier to do this using jQuery.
How to Set/Unset Cookie in jQuery
There are numerous jQuery plugins that deal with cookies. We will look at some of the simpler ones for our purpose.
1. Using jquery-cookie
jquery-cookie is a popular and free jQuery plugin that allows you to easily manipulate cookies. Here is the command to set a cookie using this plugin.
$.cookie("test", 1);
Here is the code to remove a cookie using this plugin.
$.removeCookie("test");
Here is the code to set cookie expiry to 10 days.
$.cookie("test", 1, { expires : 10 });
Here is the code to read the value of cookie.
var cookieValue = $.cookie("test");
2. Using js-cookie plugin
You can alternatively use a plain JavaScript plugin js-cookie which does not depend on jQuery. Here is the code to set cookie using this plugin.
Cookies.set('name', 'value');
Here is how to set expiry date on a cookie.
Cookies.set('name', 'value', { expires: 7 })
Here is the code to set cookie specific to given URL path.
Cookies.set('name', 'value', { expires: 7, path: '' })
Here is the code to read cookie value.
Cookies.get('name') // => 'value'
Here is the code to read all cookie values.
Cookies.get()
Here is the code to remove a cookie.
Cookies.remove('name')
If your cookie is valid to current page, then you need to supply the path parameter too.
Cookies.remove('name', { path: '' })
In this article, we have learnt how to set/unset cookie in jQuery. You can use either of these plugins to work with cookies in JavaScript.
Also read:
How to Add Days to Date in JavaScript
How to Enable Password Authentication in SSH
How to Setup SSH Keys in Ubuntu
How to Use Bash Until Loop in Linux
How to Enable Two Factor Authentication in SSH
Related posts:
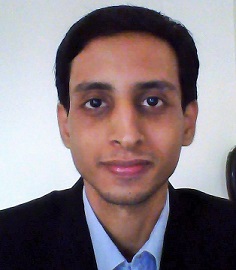
Sreeram Sreenivasan loves coding & writing tech articles. He has helped many Fortune 500 companies in the areas of BI & software development. He has more than 10 years of experience in web development, Python, Linux, SQL and database programming.