JavaScript objects are very powerful and versatile data structures that allow you to store different types of data in a compact and easy to access manner. It allows you to store data as key-value pairs that makes it easy to even convert them into JSON string and send it to web servers. Web developers need to add new property to JavaScript variables every now and then. They use literal strings as keys to store data. But sometimes you may need to add property to JavaScript object using variable, especially if you are programmatically adding property to your JavaScript object. In this article, we will learn how to add property to JavaScript object using variable.
How to Add Property to JavaScript object using Variable
Here is the syntax to add new property to JavaScript object.
object[key]=value
Let us say you have the following JavaScript object.
data = {};
Here is how we generally add new property to this JavaScript object.
data['name']='John Doe';
Now let us say you want to add new property ‘name’ using the variable ‘new_key’ then here is how to do it.
new_key = 'name'; data={ [new_key]:'John Doe' } console.log(data); // {'name':'John Doe'}
In fact, you can even use mathematical expressions as variables. Here is an example.
var data={}; var x=1; data{ [x+1]:3 }; console.log(data); //{2:3}
You can also call functions instead of using variables, as keys. Here is an example to call an imaginary function getID() of a another JavaScript object. We have also used variable to assign property value.
var data={}; data={ [someObject.getID()]: another_variable };
It is important to remember that variables can be used as property keys, only if you bracket notation in JavaScript objects. They won’t work if you use dot notation. For example, each of the following commands will give error.
var new_key='name'; var data={}; data.new_key='John Doe'; // gives error var x=1; data.x+1=3; // gives error data.someObject.getID()=another_variable; // gives error
This can be really useful for programmatically adding property to JavaScript object or modifying existing JavaScript objects.
Here is another example to add 10 properties to a JavaScript object using for loop where, in each iteration, we add new property with key=loop counter.
var data = { }; for(i = 0; i < 10; i++){ data[i] = i ; }; console.log(data); //{1:1,2:2,3:3,...,10:10}
Here also you can use variable expressions as keys, as shown below. Here we are running a loop where in each iteration we add property such that the key is a concatenation of a string an loop counter.
var data = { }; for(i = 0; i < 10; i++){ data['ab'+ i] = i ; }; console.log(data); //{'ab1':1,'ab2':2,'ab3':3,...,'ab10':10}
Of course, there are many third-party JavaScript libraries to work with objects. But for our article, we have used plain JavaScript, since you don’t really any third party libraries for this purpose. In this article, we have learnt how to add new properties to JavaScript object using variables. It is important to remember that it works only with bracket notation and not dot notation.
Also read:
How to Set/Unset Cookie in jQuery
How to Add Days to Date in JavaScript
How to Enable Password Authentication in SSH
How to Setup SSH Keys in Ubuntu
How to Use Bash Until Loop in Linux
Related posts:
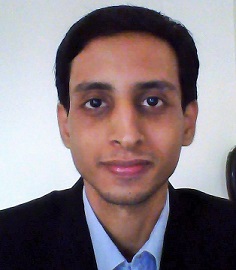
Sreeram Sreenivasan loves coding & writing tech articles. He has helped many Fortune 500 companies in the areas of BI & software development. He has more than 10 years of experience in web development, Python, Linux, SQL and database programming.