Python list is a versatile data structure that allows you to easily store different data types in a compact manner. Typically, Python developers store data in lists and iterate over them while performing different kinds of tasks. Sometimes you may need to iterate over multiple lists sequentially in Python. In this article, we will learn how to do this.
How to Iterate over Multiple Lists Sequentially in Python
There are several ways to iterate over multiple lists sequentially in Python.
1. itertools
itertools is one the best and fastest Python libraries to work with any kind of iterable data structures including lists. Let us say you have the following lists.
L1=[1,2,3]
L2=[4,5,6]
L3=[7,8,9]
You can easily iterate over these lists using itertools.chain() function. It allows you to easily work with multiple lists. Here is an example.
>>> for i in itertools.chain(L1,L2,L3):
print i
Here is the output you will see.
1
2
3
4
5
6
7
8
9
Please note, this is the fastest and most recommended way to iterate over multiple lists.
2. Using For Loop
This is the most basic and intuitive way to go through multiple lists sequentially. Let us say you have the following list of lists.
L4 = [L1, L2, L3]
print L4
[[1, 2, 3], [4, 5, 6], [7, 8, 9]]
Here is a simple nested for loop to go over list of lists.
>>> for i in L4:
for j in i:
print j
Here is the output you will see.
1
2
3
4
5
6
7
8
9
3. Using Star Operator
Python 3+ supports star(*) operator that allows you to easily unpack list of lists quickly. Here is an example to illustrate it.
L1=[1,2,3]
L2=[4,5,6]
L3=[7,8,9]
for i in [*L1, *L2, *L3]:
print(i)
Here is the output you will see.
1
2
3
4
5
6
7
8
9
In this article, we have learnt how to igo over list of lists as well as multiple lists, sequentially. Among them, using itertools.chain() is the most recommended way to do it.
Also read:
How to Check Dependencies for Package in Linux
How to Install Specific Version of NPM Package
How to Disable GZIP in Apache Server
How to Disable TLS 1.0 in Apache Server
Shell Script to Automate SSH Login
Related posts:
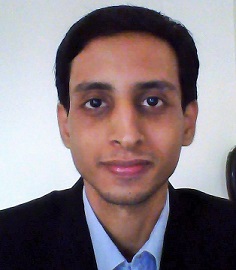
Sreeram Sreenivasan loves coding & writing tech articles. He has helped many Fortune 500 companies in the areas of BI & software development. He has more than 10 years of experience in web development, Python, Linux, SQL and database programming.