Many Python-based applications and websites need to get the current directory in order to do some processing or the other. It may be for file downloads, uploads, etc. There are several ways to easily do this in Python. In fact, the in-built os module alone is enough to retrieve current directory in Python. In this article, we will learn how to get current directory in Python, as well as how to change current directory.
How to Get Current Directory in Python
os module comes built-in in every Python installation. It provides tons of useful functions to access and work with system from within Python environment. os.getcwd() function returns the current working directory while os.chdir() function changes current working directory.
1. Using os.getcwd()
os.getcwd() stands for get current working directory. As the name implies, it returns the absolute path to current working directory.
Here is a simple example to see it in action.
import os
path = os.getcwd()
print(path)
# /home/ubuntu
print(type(path))
# <class 'str'>
In the above code, we first import os module. Then we call getwd() function and store its result in path variable. Next, we print path variable as well as its data type.
2. Using os.chdir()
os.chdir() function allows you to change current working directory. It takes one argument, the new directory to switch to. You can specify absolute path or relative path. Here is an example to illustrate it.
import os
print(os.getcwd())
# /home/ubuntu
os.chdir('../')
print(os.getcwd())
#/home
os.chdir('/tmp')
print(os.getcwd())
#/tmp
In this code, we first import os module. Next, we print our working directory ‘/home/ubuntu’. Then we change working directory the parent directory using os.chdir() function. In this case, provide relative path as the argument. Then we print the value of new current working directory.
Then we call os.chdir() function to change current working directory. This time we specify absolute path. Next, we print the current working directory.
In this article, we have learnt how to get current working directory as well as how to change this current working directory. These commands are very useful to perform file operation as well as running shell scripts from within Python environment. You can also use them to run any Linux command from within your Python application or website. The good part is that you do not need to install anything to use these functions since they are already available for free in every Python environment.
Also read:
How to Iterate over Multiple Lists in Python
How to Check Package Dependencies in Linux
How to Install Specific Version of NPM Package
How to Disable GZIP Compression in Linux
How to Disable TLS 1.0 in Apache
Related posts:
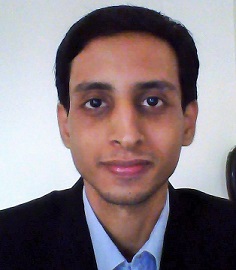
Sreeram Sreenivasan loves coding & writing tech articles. He has helped many Fortune 500 companies in the areas of BI & software development. He has more than 10 years of experience in web development, Python, Linux, SQL and database programming.