Python provides several ways to easily accept user input and process the received information. But sometimes users may enter wrong input or they may not enter any input and try to proceed further. In such cases, it is good to validate user input and ask for user input till valid response is received. In this article, we will learn how to ask for user input till valid response is received in python.
Python: Ask for User Input Till Valid Response
Python 2.x provides input() and raw_input() functions while python 3.x supports only input() function for accepting user input. For our example, we will use input() function.
Here is a simple example that asks for user input unless it receives a number.
while True: try: age = int(input("Please enter your age: ")) except ValueError: print("Please enter a number") continue else: break print age
In the above code, we run a while loop that asks for user input till it receives a number. Every user input is parsed to an integer. If the code encounters an error, it throws an exception but continues to the next iteration of the loop. If the user input is successfully parsed to an integer, it will break the loop and print the result.
You can always add more custom validation, as per your requirement. The above validation simply checks the type of user input. Here is an example where we check input with custom validation.
while True: try: age = int(input("Please enter your age: ")) except ValueError: print("Please enter a number") continue if age < 0: print("Please enter a positive number") continue else: break print age
In the above code, we first check the data type of user input, then we check if it is positive or not. Only then we break the while loop. If either of the validations fail, we simply continue to the next iteration.
Please remember to put continue statement for each validation’s failure and use break statement only if the last validation is successful.
In this article, we have seen a couple of simple ways to easily ask user input until they receive valid response.
Also read:
How to Read Input As Numbers in Python
Bash Lowercase & Uppercase Strings
How to Replace Newline With Space in Sed
How to Remove Single Quotes from Filenames in Bash
How to Reverse Git Add Before Commit
Related posts:
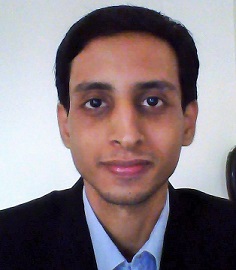
Sreeram Sreenivasan loves coding & writing tech articles. He has helped many Fortune 500 companies in the areas of BI & software development. He has more than 10 years of experience in web development, Python, Linux, SQL and database programming.