Almost every Linux user needs to work with one or more files. Linux is commonly setup as a multi-user system, with shared network folders and files. As a result, sometimes, when multiple users or processes access the same file for editing or deletion, it can lead to conflicts. In such cases, it is advisable to lock files to prevent such conflicts when multiple users or processes simultaneously access files. There are several ways to lock file in Linux. In this article, we will look at some of the common ways to lock file in Linux from editing & deleting.
How to Lock File in Linux from Editing & Deleting
When we lock a file in Linux, it prevents the file from being accessed by other processes, until the lock is released. There are two types of locks in Linux – Advisory and Mandatory Locks. We will learn how to create and release locks and how to handle errors, if any.
1. Advisory Locks
Advisory locks enables processes to request a lock on a file but does not prevent other processes from accessing it. This works in case a file needs to be accessed by multiple process such that different processes need to access different parts of the file. In this case, when a process tries to access a file that is locked, it will need to wait for the lock to be released. If it waits, it is said to be cooperating. Nevertheless, it is, however, still free to read, edit and delete the file, in which case it is said to not cooperating.
You can use fcntl() function to implement advisory locks. You can use it to lock a specific section of the file or the entire file.
Here is a simple C code to implement Advisory locks in Linux.
#include <fcntl.h> #include <stdio.h> #include <unistd.h> int main(int argc, char *argv[]) { int fd = open(argv[1], O_RDWR); struct flock fl; fl.l_type = F_WRLCK; // Set a write lock fl.l_whence = SEEK_SET; // Start at beginning of file fl.l_start = 0; // Offset from beginning of file fl.l_len = 0; // Lock entire file fl.l_pid = getpid(); // Set process ID fcntl(fd, F_SETLKW, &fl); // Set the lock printf("File locked"); sleep(10); // Sleep for 10 seconds fl.l_type = F_UNLCK; // Release the lock fcntl(fd, F_SETLK, &fl); // Unlock the file printf("File unlocked"); close(fd); return 0; }
The above code sets a lock on the file mentioned in command line argument. The fcntl() function is called with F_SETLKW flag to set the lock. After 10 seconds of sleep, it releases the lock with F_UNLCK flag.
2. Mandatory Locks
In this case, when a file is locked by a process, no other process can access the file, until the lock is released. It is set by Linux kernel and cannot be modified by other processes.
It is useful for important files that need to be prevented from other processes modifying it. Mandatory locks can also be implemented using fcntl() function. Here is a sample C code to implement it.
#include <fcntl.h> #include <stdio.h> #include <unistd.h> int main(int argc, char *argv[]) { int fd = open(argv[1], O_RDWR); struct flock fl; fl.l_type = F_WRLCK; // Set a write lock fl.l_whence = SEEK_SET; // Start at beginning of file fl.l_start = 0; // Offset from beginning of file fl.l_len = 0; // Lock entire file fl.l_pid = getpid(); // Set process ID fcntl(fd, F_SETLK, &fl); // Set the lock printf("File locked"); sleep(10); // Sleep for 10 fl.l_type = F_UNLCK; // Release the lock fcntl(fd, F_SETLK, &fl); // Unlock the file printf("File unlocked"); close(fd); return 0; }
In the above code, we use F_WRLCK flag to set the lock the file and F_UNLCK to unlock it.
At any time, you can use lslocks or cat /proc/lock commands. lslocks is available in util-linux package on all Linux systems and lists all file locks currently held in your system. On the other hand, /proc/lock is a file that holds information about all file locks in your system. We just use cat command to view its contents.
In this article, we have learnt how to lock file in Linux. You can customize it as per your requirement.
Also read:
How to Use Group By With Order By in MySQL
How to Find All .Txt Files in Directory In Python
How to Make Python Class Serializable
How to Change Sudo Timeout Period in Linux
How to Initialize JavaScript Date to Particular Time Zone
Related posts:
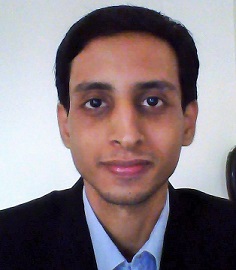
Sreeram Sreenivasan loves coding & writing tech articles. He has helped many Fortune 500 companies in the areas of BI & software development. He has more than 10 years of experience in web development, Python, Linux, SQL and database programming.